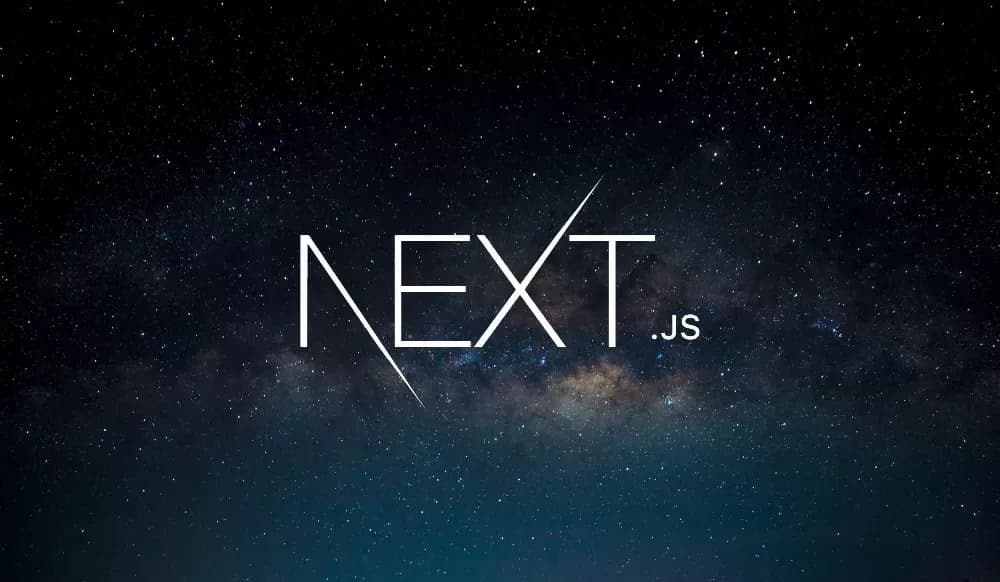
Next.js for Modern Web Development: A Comprehensive Guide
What is Next.js and Why Use It?
Next.js is a React framework that enables server-side rendering (SSR), static site generation (SSG), and incremental static regeneration (ISR). With built-in SEO optimization, fast performance, and ease of use, it's a top choice for modern web development.
Key Benefits of Next.js:
- Better SEO: Server-side rendering ensures content is indexed efficiently.
- Improved Performance: Automatic code-splitting and image optimization.
- Built-in API Routes: Develop full-stack applications effortlessly.
- Easy Deployment: Seamless integration with Vercel, the creators of Next.js.
Getting Started with Next.js
Setting up a Next.js project is straightforward. Just run:
npx create-next-app@latest my-nextjs-project
cd my-nextjs-project
npm run dev
This will start your development server on http://localhost:3000.
Essential Features of Next.js
1. Server-Side Rendering (SSR) for SEO
Using getServerSideProps, you can fetch data at request time, ensuring up-to-date content for search engines.
export async function getServerSideProps() {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return { props: { data } };
}
2. Static Site Generation (SSG) for Speed
For performance optimization, getStaticProps allows pre-building pages at build time.
export async function getStaticProps() {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return { props: { data } };
}
3. API Routes for Backend Functionality
Next.js allows you to create API endpoints within your project.
export default function handler(req, res) {
res.status(200).json({ message: 'Hello from Next.js API!' });
}
SEO Best Practices in Next.js
1. Optimizing Meta Tags
Use the built-in next/head module to manage metadata effectively.
import Head from 'next/head';
export default function Home() {
return (
<>
<Head>
<title>Next.js SEO Guide</title>
<meta name="description" content="Learn how to optimize your Next.js app for SEO." />
</Head>
<h1>Welcome to Next.js SEO Guide</h1>
</>
);
}
2. Implementing Open Graph and Twitter Cards
Enhance social media sharing with structured metadata.
<Head>
<meta property="og:title" content="Next.js SEO Guide" />
<meta property="og:description" content="Boost your Next.js site's search rankings." />
<meta property="og:image" content="/seo-image.png" />
<meta name="twitter:card" content="summary_large_image" />
</Head>
3. Using Next.js Image Optimization
Leverage the next/image component for efficient image loading and better Core Web Vitals scores.
import Image from 'next/image';
<Image src="/example.jpg" alt="Optimized image" width={600} height={400} />
Deploying Your Next.js App (Vercel)
Vercel offers an effortless deployment experience:
git push origin main
Connect your GitHub repo to Vercel and deploy instantly.
Conclusion
Next.js is a powerful framework for modern web development, offering superior SEO, performance, and developer experience. Whether you're building a personal blog or a complex web application, Next.js provides the tools to create a fast, scalable, and search-friendly website.